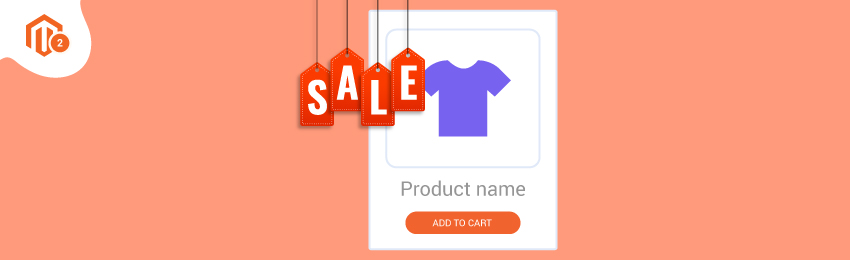
Today's tutorial will bring you various methods through which you can check if the product is on sale in Magento 2. This step-by-step tutorial will help you check if the product has a special price in Magento 2. This is required when you want to collect information about all the products that are on sale on your store.
Follow these steps:
Object Manager Method:
<?php $productId = 1; $objectManager = \Magento\Framework\App\ObjectManager::getInstance(); $product = $objectManager->create(\Magento\Catalog\Model\Product::class)->load($productId); $price = $product->getPrice(); $specialPrice = $product->getSpecialPrice(); $specialFromDate = $product->getSpecialFromDate(); $specialToDate = $product->getSpecialToDate(); $today = time(); if (!$specialPrice) { $specialPrice = $price; if ($specialPrice < $price) { if ( (is_null($specialFromDate) && is_null($specialToDate)) || ($today >= strtotime($specialFromDate) && is_null($specialToDate)) || ($today <= strtotime($specialToDate) && is_null($specialFromDate)) || ($today >= strtotime($specialFromDate) && $today <= strtotime($specialToDate)) ) { echo __('Yes. Product is on sale. Product special price is : '.$specialPrice); } else { echo __('No. Product sale has expired.'); } } } else { echo __('No. Product has not special price.'); }
Dependency Injection Method:
protected $productFactory; public function __construct( .... \Magento\Catalog\Model\ProductFactory $productFactory .... ) { .... $this->productFactory = $productFactory; .... } public function checkSpecialPriceByProId($productId) { $product = $this->productFactory->create()->load($productId); $orgprice = $product->getPrice(); $specialprice = $product->getSpecialPrice(); $specialfromdate = $product->getSpecialFromDate(); $specialtodate = $product->getSpecialToDate(); if (!$specialPrice) { $specialPrice = $price; if ($specialPrice < $price) { if ( (is_null($specialFromDate) && is_null($specialToDate)) || ($today >= strtotime($specialFromDate) && is_null($specialToDate)) || ($today <= strtotime($specialToDate) && is_null($specialFromDate)) || ($today >= strtotime($specialFromDate) && $today <= strtotime($specialToDate)) ) { echo __('Yes. Product is on sale. Product special price is : '.$specialPrice); } else { echo __('No. Product sale has expired.'); } } } else { echo __('No. Product has not special price.'); } }
You can use both methods to check the product has a special price or not. Using dependency injection you can inject class in construct and then, you can call checkSpecialPriceByProId($productId) method with the pass product ID to retrieve the output.
We hope this tutorial helped you check the products that are on sale. If we missed out anything, feel free to mention in the comments or reach us out here.
Also read: How to Add Discounted/Sale Price for Products in Magento 2?
Recommended: Magento 2 Product Label Extension to Add Custom Sales Label on Products