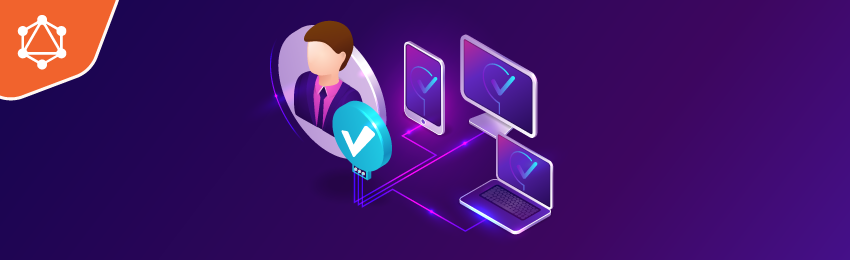
Today, we’re going to teach you how to get customer ID using graphQL in Magento 2.
GraphQL is one of the most popular features of Magento 2 that makes it easy to fetch all required data with just one request.
In simple words, by using GraphQL you can send a query to your store’s API and get what you need in response. In fact, this is the reason why most Magento 2 developers prefer using GraphQL instead of REST API.
And in this tutorial, we will show you exactly how to get customer ID using graphQL in Magento 2.
Steps to Get Customer ID using GraphQL in Magento 2
In order to get the customer ID, we need to first create a simple module to get orders’ information. And by using GraphQL, we can get data of any specific registered customer through the customer ID.
Here’s the steps you need to follow to get customer ID using GraphQL in Magento 2.
Step 1
First of all, we need to create a registration.php file in app/code/MD/CustomerGraphQL/ directory to register our module by adding the following code.
<?php \Magento\Framework\Component\ComponentRegistrar::register( \Magento\Framework\Component\ComponentRegistrar::MODULE, 'MD_CustomerGraphQL', __DIR__ );
Step 2
Now, we need to create a Module.xml file in app/code/MD/CustomerGraphQL/etc/ directory and add the following code.
This is because our module depends on GraphQL and sales module. Therefore, we have to set dependency on the Module.xml file.
<?xml version="1.0"?> <config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd"> <module name="MD_CustomerGraphQL"> <sequence> <module name="Magento_Sales"/> <module name="Magento_GraphQl"/> </sequence> </module> </config>
Now, after we’ve created the GraphQL module, we will have to contain schema.graphqls file in the etc directory of the module.
Schema.graphqls is a key file for any GraphQL query implementation and describe the data.
Step 3
The next obvious step is now to create schema.graphqls file in app/code/MD/CustomerGraphQL/etc/ directory and add the following code.
type Query { CustomerOrderList ( customer_id: Int @doc(description: "Id of the Customer") ): SalesOrder @resolver(class: "MD\\CustomerGraphQL\\Model\\Resolver\\CustomerOrder") @doc(description: "The Sales Order query returns information about a customer placed order") } type SalesOrder @doc(description: "Sales Order graphql gather data of order item information") { fetchRecords : [CustomerOrderRecord] @doc(description: "An array of customer placed order fetch records") } type CustomerOrderRecord @doc(description: "Customer placed order items information") { increment_id: String @doc(description: "Increment Id of Sales Order") customer_name: String @doc(description: "Customer name of Sales Order") grand_total: String @doc(description: "Grand total of Sales Order") qty: Int @doc(description: "Order item quantity") shipping_method: String @doc(description: "Shipping method of order placed") }
As you can see, we have passed customer_id to get the customer id as an input.
In addition, we have also created CustomerOrderRecord array to obtain sales order information, which is required in the response of the query.
Now, you can customize the field as you like.
Step 4
Lastly, we need to create CustomerOrder.php as a resolver file in app/code/MD/CustomerGraphQL/Model/Resolver/ and add the following code.
<?php declare (strict_types = 1); namespace RH\CustomerGraphQL\Model\Resolver; use Magento\Framework\Exception\NoSuchEntityException; use Magento\Framework\GraphQl\Config\Element\Field; use Magento\Framework\GraphQl\Exception\GraphQlInputException; use Magento\Framework\GraphQl\Exception\GraphQlNoSuchEntityException; use Magento\Framework\GraphQl\Query\ResolverInterface; use Magento\Framework\GraphQl\Schema\Type\ResolveInfo; class CustomerOrder implements ResolverInterface { protected $orderRepository; protected $searchCriteriaBuilder; protected $priceCurrency; public function __construct( \Magento\Sales\Api\OrderRepositoryInterface $orderRepository, \Magento\Framework\Api\SearchCriteriaBuilder $searchCriteriaBuilder, \Magento\Framework\Pricing\PriceCurrencyInterface $priceCurrency ) { $this->orderRepository = $orderRepository; $this->searchCriteriaBuilder = $searchCriteriaBuilder; $this->priceCurrency = $priceCurrency; } /** * @inheritdoc */ public function resolve( Field $field, $context, ResolveInfo $info, array $value = null, array $args = null ) { $customerId = $this->getCustomerId($args); $customerOrderData = $this->getCustomerOrderData($customerId); return $customerOrderData; } /** * @param array $args * @return int * @throws GraphQlInputException */ private function getCustomerId(array $args): int { if (!isset($args['customer_id'])) { throw new GraphQlInputException(__('Customer id should be specified')); } return (int) $args['customer_id']; } /** * @param int $customerId * @return array * @throws GraphQlNoSuchEntityException */ private function getCustomerOrderData(int $customerId): array { try { $searchCriteria = $this->searchCriteriaBuilder->addFilter('customer_id', $customerId, 'eq')->create(); $orderList = $this->orderRepository->getList($searchCriteria); $customerOrder = []; foreach ($orderList as $order) { $order_id = $order->getId(); $customerOrder['fetchRecords'][$order_id]['increment_id'] = $order->getIncrementId(); $customerOrder['fetchRecords'][$order_id]['customer_name'] = $order->getCustomerFirstname() . ' ' . $order->getCustomerLastname(); $customerOrder['fetchRecords'][$order_id]['grand_total'] = $this->priceCurrency->convertAndFormat($order->getGrandTotal(), false); $customerOrder['fetchRecords'][$order_id]['qty'] = $order->getTotalQtyOrdered(); $customerOrder['fetchRecords'][$order_id]['shipping_method'] = $order->getShippingMethod(); } } catch (NoSuchEntityException $e) { throw new GraphQlNoSuchEntityException(__($e->getMessage()), $e); } return $customerOrder; } }
And it’s done!
Now, execute the following commands:
php bin/magento s:up php bin/magento s:s:d -f php bin/magento c:c
As you can see in the output in ChromeiQL addons or in the GraphQL supported version called Postman.
Now, you need to pass query required data in Request Body.
{ CustomerOrderList (customer_id: 1) { fetchRecords{ increment_id customer_name grand_total qty shipping_method } } }
With this, we have now passed the customer ID as 1 to get the order information.
Result:
Conclusion…
And there you have it! This is how you can get customer ID using GraphQL in Magneto 2.
We hope that you found this tutorial helpful. If you have any queries, please share them in the comments below.
And if you need our professional assistance, feel free to contact us.