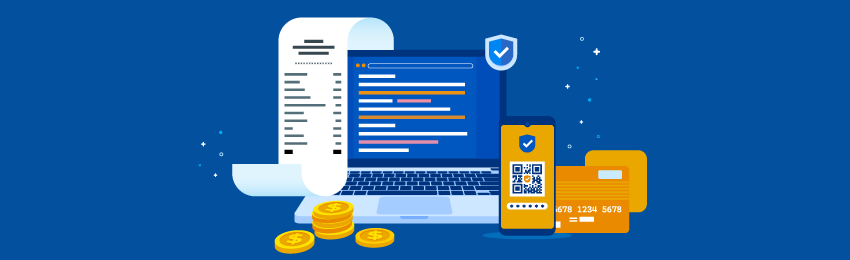
Magento 2 provides lots of commands like cache:clean, setup:upgrade, and so on to help developers with eCommerce store development.
However, there is no command for checking the available payment methods in Magento 2.
So, with this tutorial, we are going to teach you how to get the list of payment methods using the command line in Magento 2.
If you’re planning to start an eCommerce store or already have one, you will most definitely need to check payment methods.
And here, we are going to show you how to get the payment methods list programmatically.
Also read: How to Create New Command in Console CLI in Magento 2?
Now, there are 2 different ways to get the payment methods list.
- Dependency Injection (DI)
- Object Manager
Magento, however, recommends using the Dependency Injection (DI) method for fetching all payment methods list.
That being said, let’s get started.
How to Get List of Payment Methods in Magento 2 using Command Line?
Follow the below steps to get payment methods list in Magento 2 using the command line.
Step #1
Create a registration.php file at app/code/RH/CustomCommand/ and copy the following code in the file:
<?php /** * Copyright © Magento, Inc. All rights reserved. * See COPYING.txt for license details. */ \Magento\Framework\Component\ComponentRegistrar::register( \Magento\Framework\Component\ComponentRegistrar::MODULE, 'MD_CustomCommand', __DIR__ );
Step #2
Next, we need to create a module.xml file in app/code/MD/CustomCommand/etc and add the following code:
<?xml version="1.0"?> <!-- /** * Copyright © Magento, Inc. All rights reserved. * See COPYING.txt for license details. */ --> <config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd"> <module name="MD_CustomCommand" setup_version="1.0.0" schema_version="1.0.0"/> </config>
Step #3
Now, we will create a di.xml file to define the command using the following code in app/code/MD/CustomCommand/etc/ directory.
<?xml version="1.0"?> <!-- /** * Copyright © Magento, Inc. All rights reserved. * See COPYING.txt for license details. */ --> <config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:ObjectManager/etc/config.xsd"> <type name="Magento\Framework\Console\CommandList"> <arguments> <argument name="commands" xsi:type="array"> <item name="payment_list" xsi:type="object">MD\CustomCommand\Console\GetPaymentList</item> </argument> </arguments> </type> </config>
Step #4
Finally, we need to create a command class GetPaymentList.php file and copy the following code in app/code/MD/CustomCommand/Console/ directory.
<?php /** * Copyright © Magento, Inc. All rights reserved. * See COPYING.txt for license details. */ namespace MD\CustomCommand\Console; use Symfony\Component\Console\Helper\Table; use Symfony\Component\Console\Input\InputArgument; use \Symfony\Component\Console\Command\Command; use \Symfony\Component\Console\Input\InputInterface; use \Symfony\Component\Console\Output\OutputInterface; class GetPaymentList extends Command { /** * @var \Magento\Framework\App\Config\ScopeConfigInterface */ private $scopeValue; /* * @param \Magento\Framework\App\Config\ScopeConfigInterface $scopeValue [description] */ public function __construct( \Magento\Framework\App\Config\ScopeConfigInterface $scopeValue ) { $this->scopeValue = $scopeValue; parent::__construct(); } /** * {@inheritdoc} */ protected function configure() { $this->setName('info:payment:list') ->setDescription('Display Payment Method List') ->addArgument('status', InputArgument::OPTIONAL, 'Optional module name'); parent::configure(); } /** * {@inheritdoc} */ protected function execute(InputInterface $input, OutputInterface $output) { $moduleName = (string) $input->getArgument('status'); if ($moduleName == "Enabled" || $moduleName == "enabled") { return $this->showEnabledPaymentMethodList($output); }if ($moduleName == "Disabled" || $moduleName == "disabled") { return $this->showDisabledPaymentMethodList($output); }if ($moduleName == '') { return $this->showAllPaymentMethodList($output); }if ($moduleName != "" && ($moduleName != "Enabled" || $moduleName != "enabled" || $moduleName != "Disabled" || $moduleName != "disabled")) { $output->writeln('<error>Please enter correct command</error>'); return \Magento\Framework\Console\Cli::RETURN_FAILURE; } } /** * Return enabled payment method list */ private function showEnabledPaymentMethodList(OutputInterface $output) { try { $table = new Table($output); $table->setHeaders(['Code', 'Name', 'Status']); $methodList = $this->scopeValue->getValue('payment'); foreach ($methodList as $code => $_method) { $active_status = ""; $title = ""; if (isset($_method['active'])) {For display if ($_method['active'] == 1) { if (isset($_method['title'])) { $title = $_method['title']; } $table->addRow([$code, $title, 'Enable']); } } } $table->render(); return \Magento\Framework\Console\Cli::RETURN_SUCCESS; } catch (\Exception $e) { $output->writeln('<error>' . $e->getMessage() . '</error>'); if ($output->getVerbosity() >= OutputInterface::VERBOSITY_VERBOSE) { $output->writeln($e->getTraceAsString()); } return \Magento\Framework\Console\Cli::RETURN_FAILURE; } } /** * Return disabled payment method list */ private function showDisabledPaymentMethodList(OutputInterface $output) { try { $table = new Table($output); $table->setHeaders(['Code', 'Name', 'Status']); $methodList = $this->scopeValue->getValue('payment'); foreach ($methodList as $code => $_method) { $active_status = ""; $title = ""; if (isset($_method['active'])) { if ($_method['active'] == 0) { if (isset($_method['title'])) { $title = $_method['title']; } $table->addRow([$code, $title, 'Disabled']); } } } $table->render(); return \Magento\Framework\Console\Cli::RETURN_SUCCESS; } catch (\Exception $e) { $output->writeln('<error>' . $e->getMessage() . '</error>'); if ($output->getVerbosity() >= OutputInterface::VERBOSITY_VERBOSE) { $output->writeln($e->getTraceAsString()); } return \Magento\Framework\Console\Cli::RETURN_FAILURE; } } /** * Return all payment method list */ private function showAllPaymentMethodList(OutputInterface $output) { try { $table = new Table($output); $table->setHeaders(['Code', 'Name', 'Status']); $methodList = $this->scopeValue->getValue('payment'); foreach ($methodList as $code => $_method) { $active_status = ""; $title = ""; if (isset($_method['active'])) { if ($_method['active'] == 1) { $active_status = "Enable"; } else { $active_status = "Disable"; } } if (isset($_method['title'])) { $title = $_method['title']; } $table->addRow([$code, $title, $active_status]); } $table->render(); return \Magento\Framework\Console\Cli::RETURN_SUCCESS; } catch (\Exception $e) $output->writeln('<error>' . $e->getMessage() . '</error>'); if ($output->getVerbosity() >= OutputInterface::VERBOSITY_VERBOSE) { $output->writeln($e->getTraceAsString()); } return \Magento\Framework\Console\Cli::RETURN_FAILURE; } } }
And it’s done!
Now open your Command-Line in the root folder of Magento and execute the following commands:
php bin/magento s:up php bin/magento s:s:d -f php bin/magento c:c
Once you execute the above commands, you will see your custom command displayed in php bin/magento list.
This means our module is now ready to get the payment methods list.
Below, we have shared some useful commands for different requirements.
Enabled payment list only:
php bin/magento info:payment:list enabled
Disabled payment list only:
php bin/magento info:payment:list disabled
All payment list:
php bin/magento info:payment:list
Also read: Useful Magento 2 Command List for Beginners
Conclusion…
With that, we have come to the end of our tutorial on how to get list of payment methods in Magento 2.
We hope that you found this tutorial useful and in case you need further assistance, feel free to contact us for professional help.
Also read:
How to Restrict Payment Methods by Attribute/Category in Magento 2
How to Restrict Some Payment Methods in Magento 2
How to Get the Payment Method Title of an Order in Magento 2
How to Create Custom Payment Method in Magento 2
How to Setup Cash on Delivery (COD) Payment Method in Magento 2
How to Disable Payment Method for Certain Customer Groups in Magento 2