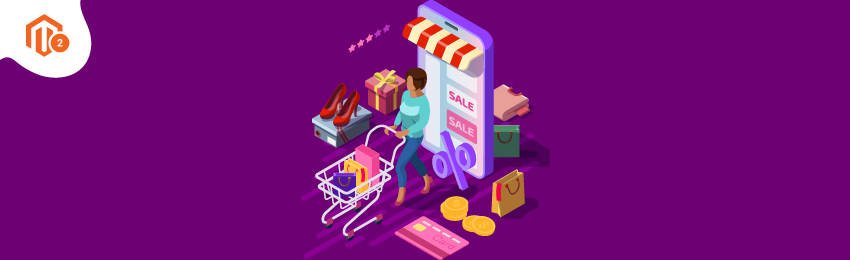
In today’s tutorial, we will help you with a detailed tutorial to get product attribute option ID from the label in Magento 2. In order to get attribute option id by the label, it is required to use \Magento\Catalog\Model\Product Factory class in the construct. A huge variety of attribute types are available such as dropdown, visual swatch, multi-select, etc wherein you can add multiple options in a single attribute.
<?php namespace MD\Helloworld\Block; class GetAttrDetails extends \Magento\Framework\View\Element\Template { /** * @var \Magento\Catalog\Model\ProductFactory */ protected $productFactory; /** * @param \Magento\Framework\View\Element\Template\Context $context * @param \Magento\Catalog\Model\ProductFactory $productFactory * @param array $data */ public function __construct( \Magento\Framework\View\Element\Template\Context $context, \Magento\Catalog\Model\ProductFactory $productFactory, array $data = [] ) { $this->productFactory = $productFactory; parent::__construct($context, $data); } /** * Pass here attribute code and option label as param */ public function getAttrOptIdByLabel($attrCode,$optLabel) { $product = $this->productFactory->create(); $isAttrExist = $product->getResource()->getAttribute($attrCode); // Add here your attribute code $optId = ''; if ($isAttrExist && $isAttrExist->usesSource()) { $optId = $isAttrExist->getSource()->getOptionId($optLabel); } return $optId; } }
This code can be used in block and model files. All you need to do is pass the attribute code and attribute option label as param in getAttrOptIdByLabel($attrCode,$optLabel) function.
This way, we can get product attribute objects from product resources and then pass option labels to retrieve attribute option id.
Now call the below-mentioned function in a template file:
$optionId = $block->getAttrOptIdByLabel('color','Red'); echo $optionId; // You will get an option ID of Red attribute option.
We hope we have covered everything in the detailed tutorial here, still if we missed anything or you get stuck anywhere while implementing, feel free to reach us out. Our experts will be happy to help.