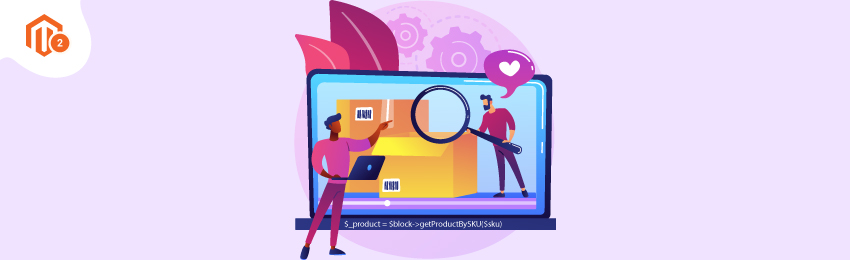
In Magento 2, getting product collection by product SKU is a very useful method when you filter the products in the catalog. In today's tutorial, we will help you how to get product collection by product SKU in Magento 2 by the below code snippet:
<?php namespace MageDelight\Training\Block; class Product extends \Magento\Framework\View\Element\Template { /** * Constructor * * @param \Magento\Framework\View\Element\Template\Context $context * @param array $data */ public function __construct( \Magento\Framework\View\Element\Template\Context $context, \Magento\Catalog\API\ProductRepositoryInterface $productRepository, array $data = [] ) { $this->productRepository = $productRepository; parent::__construct($context, $data); } /** * Get Product by SKU * @param mixed * @return \Magento\Catalog\Model\Product $product */ public function getProductBySKU($sku) { try { $product = $this->productRepository->get($sku); } catch (\Exception $exception) { throw new \Magento\Framework\Exception\NoSuchEntityException(__('Such product doesn't exist')); } return $product; } }
Now, call the function with the following code:
$sku = '24-MB01'; $product = $block->getProductBySKU($sku); echo $product->getName(); // product name echo $product->getId(); // product id
We hope we covered everything related to getting product collection by product SKU in Magento 2. If we missed out on anything, feel free to reach us out!