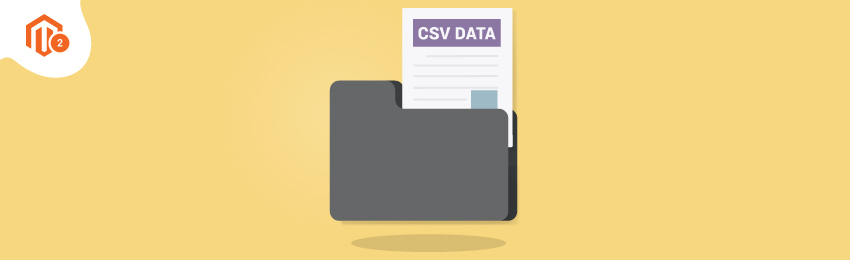
In today's tutorial, we will help you with how to read CSV data as an array in Magento 2. Developers require to read data and convert into an array to manipulate the data and make use of it in business logic.
For that, you need to use Magento\Framework\File\Csv class in your construct.
<?php namespace MD\Helloworld\Block; use Magento\Framework\Exception\FileSystemException; class ReadCsv extends \Magento\Framework\View\Element\Template { /** * @var \Magento\Framework\Filesystem\Driver\File */ protected $file; /** * @var \Magento\Framework\File\Csv */ protected $csv; /** * @var \Psr\Log\LoggerInterface */ protected $logger; /** * @param \Magento\Framework\View\Element\Template\Context $context * @param \Magento\Framework\Filesystem\Driver\File $file * @param \Magento\Framework\File\Csv $csv * @param \Psr\Log\LoggerInterface $logger * @param array $data */ public function __construct( \Magento\Framework\View\Element\Template\Context $context, \Magento\Framework\Filesystem\Driver\File $file, \Magento\Framework\File\Csv $csv, \Psr\Log\LoggerInterface $logger, array $data = [] ) { $this->file = $file; $this->csv = $csv; $this->logger = $logger; parent::__construct($context,$data); } public function getCsvData() { $data = []; $csvFile = '/var/www/html/m235/var/import/test_file.csv'; //Your CSV file path try { if ($this->file->isExists($csvFile)) { $this->csv->setDelimiter(","); $data = $this->csv->getDataPairs($csvFile); return $data; } else { $this->logger->info('csv file does not exist'); return false; } } catch (FileSystemException $e) { $this->logger->info($e->getMessage()); return false; } } }
Here, you can pass delimiter in setDelimiter() function which you want to set. Here, we use getDataPairs() function to convert CSV data into array. Post this, you can call getCsvData() function in your HTML file or anywhere and retrieve CSV file data. If you want to use this code in your controller or any other file, you are allowed to do it.
There you go!
Hope this blog has helped you make the process of reading CSV data as Array easier. Reach us out if we missed out anything.