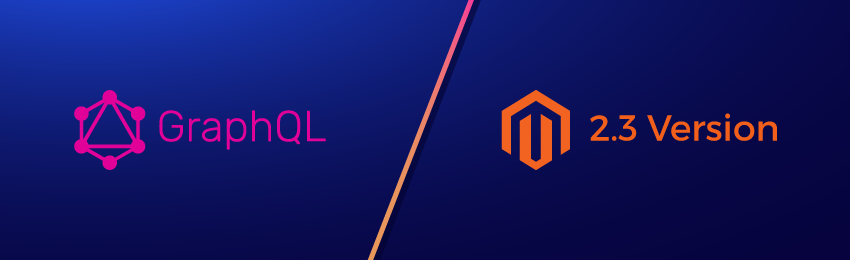
Today, we are going to teach you how to use GraphQL in Magento 2.3 version.
When Magento 2.3 was released, it came with a lot of features such as support for Progressive Web Apps (PWA), Multi-Store Inventory (MSI), GraphQL, etc.
Recommended: GraphQL & PWA Compatible Magento 2 Extensions by MageDelight
And in this tutorial, we will show you how to use GraphQL in Magento 2.3 version with a step-by-step process.
What is GraphQL?
GraphQL is basically a query language for APIs as well as for runtime to fulfill queries with your existing data.
In other words, GraphQL provides a complete description of your data in the API. And using GraphQL, you can also make a single call for retrieving all required data instead of creating several REST requests to fetch the same data.
That being said, here are a couple of key advantages of using GraphQL.
- Better performance
- Faster and Flexible
- Declarative data fetch
- Recover many resources in a single request
- No over fetching with GraphQL
Now, let’s begin with our tutorial.
Steps to use GraphQL in Magento 2.3
For implementing GraphQL, please follow the below steps.
Step 1:
First of all, we will need to create a registration.php file in app/code/MD/CustomGraphQl/ directory and add the following code.
<?php /** * Copyright © Magento, Inc. All rights reserved. * See COPYING.txt for license details. */ /** * Created By : MageDelight Pvt. Ltd. */ \Magento\Framework\Component\ComponentRegistrar::register( \Magento\Framework\Component\ComponentRegistrar::MODULE, 'MD_CustomGraphQl', __DIR__ );
Step 2:
Next, we need to create a module.xml file in app/code/MD/CustomGraphQl/etc directory and add the following code.
<?xml version="1.0"?> <!-- /** * Copyright © Magento, Inc. All rights reserved. * See COPYING.txt for license details. * * Created By : MageDelight Pvt. Ltd. */ --> <config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd"> <module name="MD_CustomGraphQl"> <sequence> <module name="Magento_GraphQl" /> </sequence> </module> </config>
The above module basically depends on the Magento_GraphQL module. Therefore, we need to set dependency in the module.xml file.
This is because when you create a GraphQL module, you’ll also need to create a schema.graphqls file in the etc directory of your module.
This file basically helps to define the structure of mutations and queries and describes which data can be used for input as well as output.
Step 3:
So, the next step is to create a schema.graphqls in the app/code/MD/CustomGraphQl/etc directory and add the following code.
# Copyright © Magento, Inc. All rights reserved. # Created By : MageDelight Pvt. Ltd. type Query { quoteData ( id: Int @doc(description: "Id of the Quote Data") ): QuoteData @resolver(class: "MD\\CustomGraphQl\\Model\\Resolver\\QuoteData") @doc(description: "The Quote Data Query Returns Data about a Quote Object") } type QuoteData @doc(description: "Quote information by quote id") { base_currency_code: String @doc(description: "Base Currency Code from Quote Object") grand_total: String @doc(description: "Grand Total of Specific Quote Object items") customer_name: String @doc(description: "Customer Name from Quote Object") }
After that, we will have to create a Resolver model for our custom module.
In that module, the resolve() method will basically return the data of quote according to the GraphQL schema type.
Step 4:
Now, we will create a QuoteData.php file in the app/code/MD/CustomGraphQl/Model/Resolver directory and add the following code.
<?php /** * Copyright © Magento, Inc. All rights reserved. * See COPYING.txt for license details. * */ /** * Created By : MageDeligt Pvt. Ltd. */ declare (strict_types = 1); namespace MD\CustomGraphQl\Model\Resolver; use Magento\Framework\Exception\NoSuchEntityException; use Magento\Framework\GraphQl\Config\Element\Field; use Magento\Framework\GraphQl\Exception\GraphQlInputException; use Magento\Framework\GraphQl\Exception\GraphQlNoSuchEntityException; use Magento\Framework\GraphQl\Query\ResolverInterface; use Magento\Framework\GraphQl\Schema\Type\ResolveInfo; /** * Quote data field resolver, used for GraphQL request processing */ class QuoteData implements ResolverInterface { /** * @var \Magento\Quote\Api\CartRepositoryInterface */ protected $quoteRepository; /** * @param \Magento\Quote\Api\CartRepositoryInterface $quoteRepository */ public function __construct( \Magento\Quote\Api\CartRepositoryInterface $quoteRepository ) { $this->quoteRepository = $quoteRepository; } /** * @inheritdoc */ public function resolve( Field $field $context, ResolveInfo $info, array $value = null array $args = null ) { $quoteId = $this->getQuoteId($args); $quoteData = $this->getQuoteData($quoteId); return $quoteData; } /** * @param array $args * @return int * @throws GraphQlNoSuchEntityException */ private function getQuoteId(array $args): int { if (!isset($args['id'])) { throw new GraphQlInputException(__('"Quote id should be specified')); } return (int) $args['id']; } /** * @param int $quoteId * @return array * @throws GraphQlNoSuchEntityException */ private function getQuoteData(int $quoteId): array { try { $quote = $this->quoteRepository->get($quoteId); $quoteData = [ 'base_currency_code' => $quote->getBaseCurrencyCode(), 'grand_total' => $quote->getGrandTotal(), 'customer_name' => $quote->getCustomerFirstname() . ' ' . $quote->getCustomerLastname(), ]; } catch (NoSuchEntityException $e) { throw new GraphQlNoSuchEntityException(__($e->getMessage()), $e); } return $quoteData; } }
And it’s done!
Now, we need to run the following commands to install our custom module:
php bin/magento s:up php bin/magento s:s:d -f php bin/magento c:c
Lastly, it’s time to check query output by either installing Postman (GraphQL supported version) or by installing Google Chrome extension ChromeiQL.
After installing any one of the above, execute the following URL:
http://127.0.0.1/m231/graphql
After that, you will need to pass the following data where your id is your quote id in Request Body.
{ quoteData(id: 1) { base_currency_code customer_name grand_total } }
Output:
{ "data": { "quoteData": { "base_currency_code": "USD", "grand_total": "36.3900", "customer_name": "Veronica Costello" } } }
Conclusion
With this, we have come to the end of this post. We hope that you found this tutorial helpful. If you have any questions, please share them in the comments.
And if you need our professional assistance, feel free to contact us at any time.